Let’s understand Multidimensional array with real life scenario. Suppose you went to buy ticket on ticket counter, it may be movie ticket, rail ticket or bus ticket there are two lines mentioned. One is for male and second one is for female. If there is only one line, then it is called as one dimension. As there is more than one line, so it may be called as multi dimension (2 dimension in this case). So if there are multiple rows and multiple columns, then it is called as Multi-dimension. You can think it like a spreadsheet because there are number of rows and columns available on the sheet.
Array is continuous memory locations used to store homogenous data means a data of similar type and MultiDimensional Array is used to store the values in the rows as well as in columns.
Important Note: Java does not support multidimensional array. Multidimensional array in java is basically “array of arrays”.
Table of Contents
Multidimensional Array Explanation:
To understand the basic structure of multidimensional just focus on below image.
Position of multidimensional array of [6][4] size:
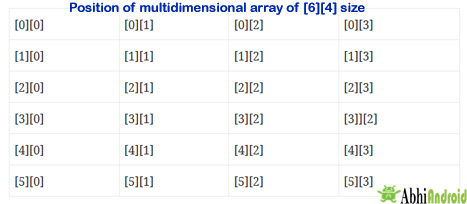
Another real life example you can consider is Egg tray in which eggs are stored in rows and columns.
Important Note: Multidimensional array basically depend on the number of subscripts used. If you use two, it is called as 2D, if you use three then it is called 3D and if you use four then it is called 4D and so on. Here D stands for Dimensions.
Multidimensional Array Declaration in Java:
If we want to declare an array having 2 rows and 3 columns. Then it can be written as
int[][] twoDimensionalArray= new int[2][3];
Suppose two index values are given. But, If you want to make second one empty (i.e. null without value) you can do that. But first one is mandatory to specify. Otherwise you will get an compile time error.
int[][] twoDimensionalArray= new int[2][];//right way to write int[][] twoDimensionalArray= new int[][3];//wrong way to write
Important Note: As already discussed that multidimensional array is array of arrays. So you can specify your array for second subscript. But it should not exceed the your first index value otherwise you will get array out of bound exception.
Important Note 2: Array of size [2][] means its having two rows and column can be equal or less than two.
Right pattern to code:
//Right pattern of code int[][] twoDArray=new int[2][]; twoDArray[0]=new int[2]; twoDArray[1]=new int[2];
Wrong pattern to code
//Wrong pattern of code int[][] twoDArray=new int[2][]; twoDArray[0]=new int[3]; twoDArray[1]=new int[4];
String Array Declaration:
Same pattern is applied if you want to declare a multidimensional array of strings. It can be written as:
String[][] str1= new String[2][2]; //String Declaration
Multidimensional Array Initialization in Java:
To initialize an array means, providing value to this array at proper position after its declaration.
/*2D integer Array defined here can have two rows and column can be equal or less than two*/ int[][] twoDArray=new int[2][]; twoDArray[0]=new int[2]; twoDArray[1]=new int[2]; /*value assigned are 1,2,4,5 at [0][0],[0][1],[1][0],[1][1] index values respectively*/ twoDArray[0][0]=1; twoDArray[0][1]=2; twoDArray[1][0]=4; twoDArray[1][1]=5;
String initialization in multidimensional array:
String[][] str1= new String[2][2]; /*String array is used to store four different string. And stored values are: Hello Mr How You*/ str1[0][0]="Hello"; str1[0][1]="Mr"; str1[1][0]="How"; str1[1][1]="You";
Multidimensional Array Example Program:
All we have discussed till there, everything is merged in single program to help you understand the concept about Multidimensional array.
Important Note: We have considered the use of for loop because its mandatory to traverse the elements in the array using loop. So we consider two for loops for each array. Outer for loop is for rows and inner for loop is for columns. And that will be pretty clear to see the output given for this program just below the program.
Program for MultiDimensional Array in java
public class MultiDimensionalArray{ /**Program For MultiDimensional Array in java * @param args */ public static void main(String[] args) { int[][] twoDimensionalArray= new int[2][3]; int[][] twoDArray=new int[2][]; twoDArray[0]=new int[2]; twoDArray[1]=new int[2]; twoDArray[0][0]=1; twoDArray[0][1]=2; twoDArray[1][0]=4; twoDArray[1][1]=5; String[][] str={{"Hello","Mr"},{"How","You"}}; String[][] str1= new String[2][2]; str1[0][0]="Hello"; str1[0][1]="Mr"; str1[1][0]="How"; str1[1][1]="You"; System.out.println("The Result for twodimenionalarray is:"); twoDimensionalArray[0][0]=0; for(int i=0;i<2;i++){ for(int j=0; j<3; j++){ twoDimensionalArray[i][j]=i+j; System.out.print(+twoDimensionalArray[i][j]); System.out.print("\t"); } System.out.print("\n"); } System.out.println("The Result for twoDArray is:"); for(int i=0;i<2;i++){ for(int j=0; j<2; j++){ System.out.print(twoDArray[i][j]); System.out.print("\t"); } System.out.print("\n"); } System.out.println("The Result for String Array is:"); for(int i=0;i<2;i++){ for(int j=0; j<2; j++){ System.out.print(str[i][j]); System.out.print("\t"); } System.out.print("\n"); } System.out.println("The Result for String Array by initializing in different way is:"); for(int i=0;i<2;i++){ for(int j=0; j<2; j++){ System.out.print(str[i][j]); System.out.print("\t"); } System.out.print("\n"); } } }
Output of program for Multi-Dimesional Array in java:
The Result for twodimenionalarray is: 0 1 2 1 2 3 The Result for twoDArray is: 1 2 4 5 The Result for String Array is: Hello Mr How You The Result for String Array by initializing in different way is: Hello Mr How You
Applications/Use of Multidimensional Array in Real Life:
If you think, there are many game application where you can use Multi-dimensional array. If we talk about game then chess is first game where rows and columns are used. Second is tic-toe game and other board games are there too. Apart from games these can be used in matrix calculations and lots of other areas depending on requirement.
Key Points To Remember About Multidimensional Array:
- These are very fast in access. Because in these values are traverse through the indexes.
- Insertion and deletion is very costly in between because all the elements traverse or move either forward or backward.
- Its size is fixed. So cannot move beyond the limits.
- These are helpful where you know the exact size of assignments.