Table Of Contents
onStart()
- When activity start getting visible to user then onStart() will be called.
- This calls just after the onCreate() at first time launch of activity.
- When activity launch, first onCreate() method call then onStart() and then onResume().
- If the activity is in onPause() condition i.e. not visible to user. And if user again launch the activity then onStart() method will be called.
onStart() Example In Android:
Lets create a simple program in Android that will show a message on screen when onStart() method will be called. We will use Toast class to show up the message on screen.
First create a new project, name activity as MainActivity and create a content_main.xml in layout folder if not present by default.
The UI will be very simple as we have just one TextView which say onStart() call after onCreate().
Below is the code of content_main.xml
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="fill_parent" android:layout_height="fill_parent"> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:textAppearance="?android:attr/textAppearanceMedium" android:text="onStart() Call after onCreate()" /> </RelativeLayout>
We have used Toast class to display message when onCreate() and onStart() is called.
Below is the code of MainActivity.java
package abhiandroid.com.exampleonstart; import android.os.Bundle; import android.support.design.widget.FloatingActionButton; import android.support.design.widget.Snackbar; import android.support.v7.app.AppCompatActivity; import android.support.v7.widget.Toolbar; import android.view.View; import android.view.Menu; import android.view.MenuItem; import android.widget.Toast; public class MainActivity extends AppCompatActivity { @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.content_main); Toast.makeText(getApplicationContext(), "First onCreate() calls", Toast.LENGTH_SHORT).show(); //onCreate called } @Override protected void onStart() { super.onStart(); Toast.makeText(getApplicationContext(),"Now onStart() calls", Toast.LENGTH_LONG).show(); //onStart Called } }
Output:
Now runs the program in AVD Emulator. You will see two Toast messages showing first onCreate() method is called and then onStart():
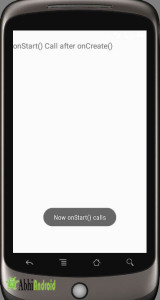
on resume is only called after onpause and then again onstart i tthink
There is a room for a better explanation to your demo above, if you add onPause, onStop method and then upload may be a video probably couple of seconds long showing the transitions of Activity Lifecycle, and Toast message changing accordingly.